Angular 11 - HTTP Client Quickstart Guide
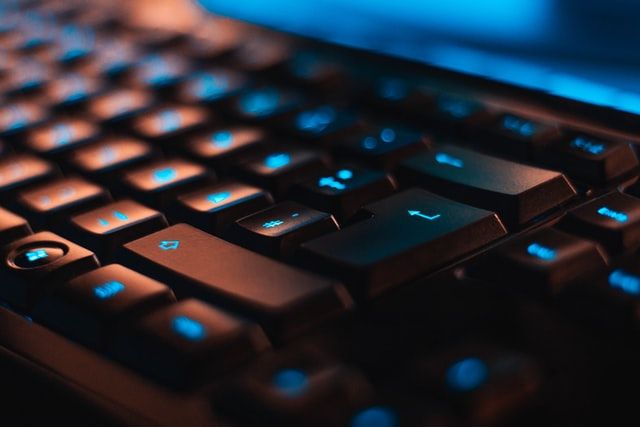
This tutorial will walk you through the Angular 11 HTTP Client module in a step-by-step manner. In general, we'll go over how to use HTTP in Angular. I'll show you how to make HTTP requests from your Angular 11 application to a backend server.
Prerequisites
Before we continue we will need the following:
- Angular CLI 11 installed.
- Basic knowledge in JavaScript and Angular
- HTTP Requests knowledge
Getting Started
This tutorial assumes that you have the Angular 11 application installed in your local machine.
Now head over to your Angular application root directory by running the following command:
cd my-app && ng start // starts angular application
Next, open app.module.ts
in your text editor and import the angular HTTP client.
import { NgModule } from '@angular/core';
import { HttpClientModule } from '@angular/common/http';
@NgModule({
declarations: [
AppComponent
],
imports: [
HttpClientModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
With the HTTP module in our application, let's proceed and implement requests to external servers in our app.component.ts
component that's generated by the Angular CLI by default.
Open your app.component.ts
and add the following scripts:
import { Component, OnInit } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'my-app', //
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
constructor(private httpClient: HttpClient) { }
ngOnInit(): void {}
}
In the above script, we've imported HttpClient and injected it into our class' constructor. This is known as dependency injection.
Dependency injection, simplified as DI is a mechanism by which the AppComponent class will be able to use HTTPClient dependencies.
HTTP GET requests
Now that we've injected HTTP into our class, the next step involves making requests to some servers.
The invoked server should respond to our requests and return some data.
We can then use this data in our application.
In our app.component.ts
script file, add the following just below the ngonInit(){}
method.
getAllUsers() {
this.HttpClient.get<User[]>('https://www.myapiurl.com/users')
.subscribe(response => {
console.log(response);
},
error => {
console.log(error);
}
);
}
In the script above, we've created a method getAllUsers()
. This method has an HTTP method used to fetch users from an endpoint 'https://www.myapiurl.com/users
. The GET method is expecting an array of users as typed just before the endpoint GET<Users[]>
.
You've noticed we subscribed to API response. This feature is known as observable in JavaScript, which is used to manage async data.
The response we subscribed to are logged in our console. To use this response in our template let's assign it to a variable.
Let's create a variable users
in our class and assign it the response from our API.
import { Component, OnInit } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'my-app', //
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
users: User =[];
constructor(private httpClient: HttpClient) { }
ngOnInit(): void
{this.getAllusers(); // this is called on page load}
getAllUsers() {
this.HttpClient.get<User[]>('https://www.myapiurl.com/users')
.subscribe(response => {
this.users = response;
},
error => {
console.log(error);
}
);
}
}
Now let's head over to our app.component.html
and display users in our browser.
<table class='table'>
<thead>
<tr>
<th>First Name</th>
<th>Last Name</th>
<th>Username</th>
<th>Email</th>
<th>Phone Number</th>
<th>Address</th>
</tr>
</thead>
<tbody>
<tr *ngFor ="let user of users">
<td>{{user.first_name}}</td>
<td>{{user.last_name}}</td>
<td>{{user.username}}</td>
<td>{{user.email}}</td>
<td>{{user.phone_number}}</td>
<td>{{user.address}}</td>
</tr>
</tbody>
</table>
You may have noticed that we used for..loop
in our template. In our script, we defined our GET method to return an array of users, to get a single response, we have to run a loop.
It's that simple to make HTTP GET requests in Angular.
HTTP POST requests
We've seen how to make GET requests, let's see how we can make POST requests to an external web service.
In your app.component.ts
script, add the following method that we'll use to send data.
import { Component, OnInit } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { FormBuilder, FormGroup, validators} from '@angular/forms';
@Component({
selector: 'my-app', //
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
users: User =[];
registerUserForm: FormGroup;
constructor(private httpClient: HttpClient, private formBuilder: FormBuilder) { }
ngOnInit(): void
{
this.register(); // this is called on page load
this.registerUserForm = this.formBuilder.group({
first_name : [''],
last_name: [''],
username: [''],
email: ['', Validators.email]
phone: [''],
address: [''],
});
}
register() {
this.HttpClient.post<User[]>('https://www.myapiurl.com/users', this.registeruserForm.value)
.subscribe(response => {
this.users = response;
},
error => {
console.log(error);
}
);
}
}
This script has a Forms module that is used to interact with the user. We capture this data and submit it to our API via the register() user method. The POST method takes two parameters, the endpoint URL and the data we're sending, in our case user authentication details.
We can then proceed to listen to the response returned by the API.
Summary
We've seen how we can make requests to external web services and how to use these data. You can proceed and make requests with the PUT, DELETE, and PATCH methods.
Happy coding.
Peer review contribution by: Odhiambo Paul
